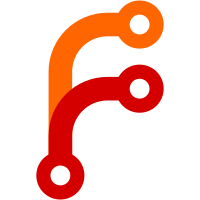
This converts os_snprintf() result validation cases to use os_snprintf_error() where the exact rule used in os_snprintf_error() was used. These changes were done automatically with spatch using the following semantic patch: @@ identifier E1; expression E2,E3,E4,E5,E6; statement S1; @@ ( E1 = os_snprintf(E2, E3, ...); | int E1 = os_snprintf(E2, E3, ...); | if (E5) E1 = os_snprintf(E2, E3, ...); else E1 = os_snprintf(E2, E3, ...); | if (E5) E1 = os_snprintf(E2, E3, ...); else if (E6) E1 = os_snprintf(E2, E3, ...); else E1 = 0; | if (E5) { ... E1 = os_snprintf(E2, E3, ...); } else { ... return -1; } | if (E5) { ... E1 = os_snprintf(E2, E3, ...); } else if (E6) { ... E1 = os_snprintf(E2, E3, ...); } else { ... return -1; } | if (E5) { ... E1 = os_snprintf(E2, E3, ...); } else { ... E1 = os_snprintf(E2, E3, ...); } ) ? os_free(E4); - if (E1 < 0 || \( E1 >= E3 \| (size_t) E1 >= E3 \| (unsigned int) E1 >= E3 \| E1 >= (int) E3 \)) + if (os_snprintf_error(E3, E1)) ( S1 | { ... } ) Signed-off-by: Jouni Malinen <j@w1.fi>
71 lines
1.3 KiB
C
71 lines
1.3 KiB
C
/*
|
|
* Universally Unique IDentifier (UUID)
|
|
* Copyright (c) 2008, Jouni Malinen <j@w1.fi>
|
|
*
|
|
* This software may be distributed under the terms of the BSD license.
|
|
* See README for more details.
|
|
*/
|
|
|
|
#include "includes.h"
|
|
|
|
#include "common.h"
|
|
#include "uuid.h"
|
|
|
|
int uuid_str2bin(const char *str, u8 *bin)
|
|
{
|
|
const char *pos;
|
|
u8 *opos;
|
|
|
|
pos = str;
|
|
opos = bin;
|
|
|
|
if (hexstr2bin(pos, opos, 4))
|
|
return -1;
|
|
pos += 8;
|
|
opos += 4;
|
|
|
|
if (*pos++ != '-' || hexstr2bin(pos, opos, 2))
|
|
return -1;
|
|
pos += 4;
|
|
opos += 2;
|
|
|
|
if (*pos++ != '-' || hexstr2bin(pos, opos, 2))
|
|
return -1;
|
|
pos += 4;
|
|
opos += 2;
|
|
|
|
if (*pos++ != '-' || hexstr2bin(pos, opos, 2))
|
|
return -1;
|
|
pos += 4;
|
|
opos += 2;
|
|
|
|
if (*pos++ != '-' || hexstr2bin(pos, opos, 6))
|
|
return -1;
|
|
|
|
return 0;
|
|
}
|
|
|
|
|
|
int uuid_bin2str(const u8 *bin, char *str, size_t max_len)
|
|
{
|
|
int len;
|
|
len = os_snprintf(str, max_len, "%02x%02x%02x%02x-%02x%02x-%02x%02x-"
|
|
"%02x%02x-%02x%02x%02x%02x%02x%02x",
|
|
bin[0], bin[1], bin[2], bin[3],
|
|
bin[4], bin[5], bin[6], bin[7],
|
|
bin[8], bin[9], bin[10], bin[11],
|
|
bin[12], bin[13], bin[14], bin[15]);
|
|
if (os_snprintf_error(max_len, len))
|
|
return -1;
|
|
return 0;
|
|
}
|
|
|
|
|
|
int is_nil_uuid(const u8 *uuid)
|
|
{
|
|
int i;
|
|
for (i = 0; i < UUID_LEN; i++)
|
|
if (uuid[i])
|
|
return 0;
|
|
return 1;
|
|
}
|