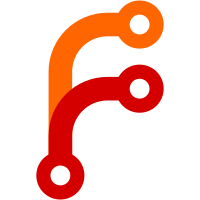
The rcons[] and Td4s[] array values need to be type cast explicitly to u32 for the left shift 24 operation to be defined due to the implicit conversion to int not handling the case where MSB would become 1 without depending on UB. Credit to OSS-Fuzz: https://bugs.chromium.org/p/oss-fuzz/issues/detail?id=14929 Signed-off-by: Jouni Malinen <jouni@codeaurora.org>
126 lines
4.2 KiB
C
126 lines
4.2 KiB
C
/*
|
|
* AES (Rijndael) cipher
|
|
* Copyright (c) 2003-2012, Jouni Malinen <j@w1.fi>
|
|
*
|
|
* This software may be distributed under the terms of the BSD license.
|
|
* See README for more details.
|
|
*/
|
|
|
|
#ifndef AES_I_H
|
|
#define AES_I_H
|
|
|
|
#include "aes.h"
|
|
|
|
/* #define FULL_UNROLL */
|
|
#define AES_SMALL_TABLES
|
|
|
|
extern const u32 Te0[256];
|
|
extern const u32 Te1[256];
|
|
extern const u32 Te2[256];
|
|
extern const u32 Te3[256];
|
|
extern const u32 Te4[256];
|
|
extern const u32 Td0[256];
|
|
extern const u32 Td1[256];
|
|
extern const u32 Td2[256];
|
|
extern const u32 Td3[256];
|
|
extern const u32 Td4[256];
|
|
extern const u32 rcon[10];
|
|
extern const u8 Td4s[256];
|
|
extern const u8 rcons[10];
|
|
|
|
#ifndef AES_SMALL_TABLES
|
|
|
|
#define RCON(i) rcon[(i)]
|
|
|
|
#define TE0(i) Te0[((i) >> 24) & 0xff]
|
|
#define TE1(i) Te1[((i) >> 16) & 0xff]
|
|
#define TE2(i) Te2[((i) >> 8) & 0xff]
|
|
#define TE3(i) Te3[(i) & 0xff]
|
|
#define TE41(i) (Te4[((i) >> 24) & 0xff] & 0xff000000)
|
|
#define TE42(i) (Te4[((i) >> 16) & 0xff] & 0x00ff0000)
|
|
#define TE43(i) (Te4[((i) >> 8) & 0xff] & 0x0000ff00)
|
|
#define TE44(i) (Te4[(i) & 0xff] & 0x000000ff)
|
|
#define TE421(i) (Te4[((i) >> 16) & 0xff] & 0xff000000)
|
|
#define TE432(i) (Te4[((i) >> 8) & 0xff] & 0x00ff0000)
|
|
#define TE443(i) (Te4[(i) & 0xff] & 0x0000ff00)
|
|
#define TE414(i) (Te4[((i) >> 24) & 0xff] & 0x000000ff)
|
|
#define TE411(i) (Te4[((i) >> 24) & 0xff] & 0xff000000)
|
|
#define TE422(i) (Te4[((i) >> 16) & 0xff] & 0x00ff0000)
|
|
#define TE433(i) (Te4[((i) >> 8) & 0xff] & 0x0000ff00)
|
|
#define TE444(i) (Te4[(i) & 0xff] & 0x000000ff)
|
|
#define TE4(i) (Te4[(i)] & 0x000000ff)
|
|
|
|
#define TD0(i) Td0[((i) >> 24) & 0xff]
|
|
#define TD1(i) Td1[((i) >> 16) & 0xff]
|
|
#define TD2(i) Td2[((i) >> 8) & 0xff]
|
|
#define TD3(i) Td3[(i) & 0xff]
|
|
#define TD41(i) (Td4[((i) >> 24) & 0xff] & 0xff000000)
|
|
#define TD42(i) (Td4[((i) >> 16) & 0xff] & 0x00ff0000)
|
|
#define TD43(i) (Td4[((i) >> 8) & 0xff] & 0x0000ff00)
|
|
#define TD44(i) (Td4[(i) & 0xff] & 0x000000ff)
|
|
#define TD0_(i) Td0[(i) & 0xff]
|
|
#define TD1_(i) Td1[(i) & 0xff]
|
|
#define TD2_(i) Td2[(i) & 0xff]
|
|
#define TD3_(i) Td3[(i) & 0xff]
|
|
|
|
#else /* AES_SMALL_TABLES */
|
|
|
|
#define RCON(i) ((u32) rcons[(i)] << 24)
|
|
|
|
static inline u32 rotr(u32 val, int bits)
|
|
{
|
|
return (val >> bits) | (val << (32 - bits));
|
|
}
|
|
|
|
#define TE0(i) Te0[((i) >> 24) & 0xff]
|
|
#define TE1(i) rotr(Te0[((i) >> 16) & 0xff], 8)
|
|
#define TE2(i) rotr(Te0[((i) >> 8) & 0xff], 16)
|
|
#define TE3(i) rotr(Te0[(i) & 0xff], 24)
|
|
#define TE41(i) ((Te0[((i) >> 24) & 0xff] << 8) & 0xff000000)
|
|
#define TE42(i) (Te0[((i) >> 16) & 0xff] & 0x00ff0000)
|
|
#define TE43(i) (Te0[((i) >> 8) & 0xff] & 0x0000ff00)
|
|
#define TE44(i) ((Te0[(i) & 0xff] >> 8) & 0x000000ff)
|
|
#define TE421(i) ((Te0[((i) >> 16) & 0xff] << 8) & 0xff000000)
|
|
#define TE432(i) (Te0[((i) >> 8) & 0xff] & 0x00ff0000)
|
|
#define TE443(i) (Te0[(i) & 0xff] & 0x0000ff00)
|
|
#define TE414(i) ((Te0[((i) >> 24) & 0xff] >> 8) & 0x000000ff)
|
|
#define TE411(i) ((Te0[((i) >> 24) & 0xff] << 8) & 0xff000000)
|
|
#define TE422(i) (Te0[((i) >> 16) & 0xff] & 0x00ff0000)
|
|
#define TE433(i) (Te0[((i) >> 8) & 0xff] & 0x0000ff00)
|
|
#define TE444(i) ((Te0[(i) & 0xff] >> 8) & 0x000000ff)
|
|
#define TE4(i) ((Te0[(i)] >> 8) & 0x000000ff)
|
|
|
|
#define TD0(i) Td0[((i) >> 24) & 0xff]
|
|
#define TD1(i) rotr(Td0[((i) >> 16) & 0xff], 8)
|
|
#define TD2(i) rotr(Td0[((i) >> 8) & 0xff], 16)
|
|
#define TD3(i) rotr(Td0[(i) & 0xff], 24)
|
|
#define TD41(i) ((u32) Td4s[((i) >> 24) & 0xff] << 24)
|
|
#define TD42(i) ((u32) Td4s[((i) >> 16) & 0xff] << 16)
|
|
#define TD43(i) ((u32) Td4s[((i) >> 8) & 0xff] << 8)
|
|
#define TD44(i) ((u32) Td4s[(i) & 0xff])
|
|
#define TD0_(i) Td0[(i) & 0xff]
|
|
#define TD1_(i) rotr(Td0[(i) & 0xff], 8)
|
|
#define TD2_(i) rotr(Td0[(i) & 0xff], 16)
|
|
#define TD3_(i) rotr(Td0[(i) & 0xff], 24)
|
|
|
|
#endif /* AES_SMALL_TABLES */
|
|
|
|
#ifdef _MSC_VER
|
|
#define SWAP(x) (_lrotl(x, 8) & 0x00ff00ff | _lrotr(x, 8) & 0xff00ff00)
|
|
#define GETU32(p) SWAP(*((u32 *)(p)))
|
|
#define PUTU32(ct, st) { *((u32 *)(ct)) = SWAP((st)); }
|
|
#else
|
|
#define GETU32(pt) (((u32)(pt)[0] << 24) ^ ((u32)(pt)[1] << 16) ^ \
|
|
((u32)(pt)[2] << 8) ^ ((u32)(pt)[3]))
|
|
#define PUTU32(ct, st) { \
|
|
(ct)[0] = (u8)((st) >> 24); (ct)[1] = (u8)((st) >> 16); \
|
|
(ct)[2] = (u8)((st) >> 8); (ct)[3] = (u8)(st); }
|
|
#endif
|
|
|
|
#define AES_PRIV_SIZE (4 * 4 * 15 + 4)
|
|
#define AES_PRIV_NR_POS (4 * 15)
|
|
|
|
int rijndaelKeySetupEnc(u32 rk[], const u8 cipherKey[], int keyBits);
|
|
|
|
#endif /* AES_I_H */
|